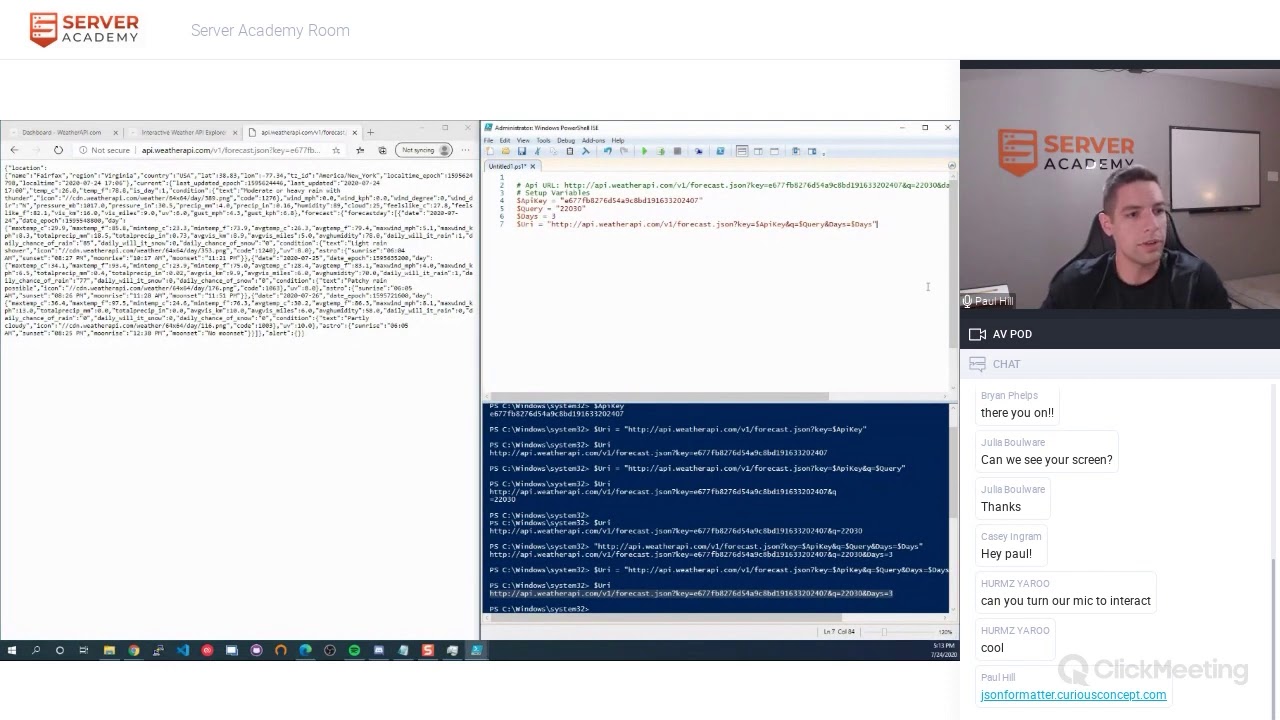
PowerShell Web Requests (APIs)
In this live training session you are going to learn how to use PowerShell to initiate web requests and manipulate data through APIs.
Downloads:
IT Lab
# https://www.weatherapi.com/pricing.aspx
# Sign up for the free vesion
# Confirm your email and log in
# Regenerate your API key
$ApiKey = "e677fb8276d54a9c8bd191633202407"
$Query = "22030"
$Days = 7
$Uri = "http://api.weatherapi.com/v1/forecast.json?key=$ApiKey&q=$Query&Days=$Days"
# Output the URI we will be making a call to
Write-Host "API call Uri: $Uri"
# You can copy this into your web browser and view the result
# You can paste the result into a JSON formatter for easier visibility
# https://jsonformatter.curiousconcept.com/
# Make the request
if(!$Request) { $Request = Invoke-WebRequest -Uri $Uri } else { write-host "Not initiating API call since we already have the data" }
# Get the content
$Content = $Request.Content
# Convert from JSON
$ConvertedJson = $Request.Content | ConvertFrom-Json
## For PowerShell 6 and above run the following command:
# $ConvertedJsonHash = $Request.Content | ConvertFrom-Json -AsHashtable
# Expand the JSON objecct
$ConvertedFormattedJson = $Request.Content | ConvertFrom-Json | Format-List
# Other formatting options:
# Format-Custom
# Format-Hex
# Format-List
# Format-Table
# Format-Wide
# Cannot access data inside of formated object
# The following line returns empty
$ConvertedFormattedJson.location
# Grab the location information
$ConvertedJson.location
$ConvertedJson.location.name
$ConvertedJson.location.region
$ConvertedJson.location.country
# Grab the forecast for the next couple days
$Forecast = $ConvertedJson.forecast.forecastday
# Iterate over the forecast and output the data
Foreach($Day in $Forecast) {
# Prep variables
$Date = $Day.date
$High = $Day.Day.maxtemp_f
$Low = $Day.Day.mintemp_f
$Condition = $day.day.condition.text
# Output forecast
Write-host "$Date will have a high of $High and a low of $Low and will be $Condition"
}
# # Change the formatting on the date
Foreach($Day in $Forecast) {
# Prep variables
$Date = $Day.date | get-date -Format "dddd"
$High = $Day.Day.maxtemp_f
$Low = $Day.Day.mintemp_f
$Condition = $day.day.condition.text
# Output forecast
Write-host "$Date will have a high of $High and a low of $Low and will be $Condition"
}